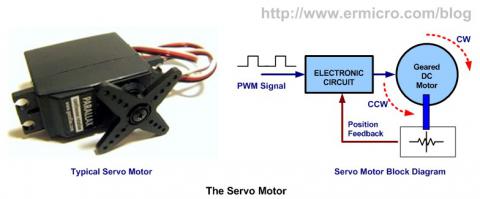
DC motor is very simple to operate, you power it and it rotates in one direction and if you change the polarity of the power it rotates in the opposite directions
Advantages of DC motor
- Low cost
- Easy to get going, all you need is a power supply
- High RPM ( High speed )
Disadvantages
- Low Torque: Not practical to use it the drive a toy without gears
- Position control is not possible
- Low speeds are difficult to achieve
Servos, have few advantages over DC motors:
- High Torque
- Low speed
- Precision control
One problem with servos is that they cost a lot more than DC motors.
How to convert a DC motor to a Servo motor:
The basic idea behind converting a DC motor to servo is to find the position of the shaft and apply a DC voltage to get the Shaft to the expected position.
The below diagram illustrates the idea:
Controlling a DC motor in Servo mode:
Items required
- Mega or Uno
- L293 Motor controller
- DC motor
- Potentiometer
Ref: http://www.instructables.com/id/DIY-Servo-Motor/
//Arduino code to control angle of a motor shaft using a potentiometer for feedback
//Please use a low rpm motor. Not more than about 500 rpm.
//******POTENTIOMETER SETUP *************
//1. Fix the shaft of the potentiometer to the motor shaft.You might like to use a slightly flexible coupling
//to do this, otherwise even a slight misalignment may cause trouble.
//2. Fix the body of the potentiometer to a rigid surface such as the body of the motor,//so that when the motor shaft turns, only the potentiometer shaft turns with it.
//3. Now we can read the potentiometer value to get the angle of the motor shaft//Look at my youtube video to see how I did this. In my video, I fixed the BODY of the potentiometer//to the motor shaft. It will be better to fix the SHAFT of the potentiomter to the motor
//shaft if you can do it properly
//Fix santa's hand to the motor shaft so that it does not interfere with the potentiometer movement
intpotPin=5;
//we will read the potentiometer value on analog pin 5
//**********************************************
//*****ANGULAR CONSTANTS********
#definePOT_VALUE_MAX700
// potentiometer reading when motor shaft is at 180 degree position.
//You will need to fill this value according to your setup.See below....
#definePOT_VALUE_MIN200
//potentiometer reading when when motor shaft is at 0 degree position.
//You will need to fill this value according to your setup.See below....
//To fill up the correct values, first turn the motor shaft manually to 0 degree position.
//Now read the potentiometer value and edit the #define POT_VALUE_MIN line with your pot reading.
//Next manually move the motor shaft to 180 degree position,
//read the pot value and edit #define POT_VALUE_MAX line with your pot reading.
#definePERM_ERROR3//the max permissible error in degrees. In my potentiometer, a turn only about 3 degrees
//on the potentiometer shaft causes any real change in the ohmic reading. You can adjust this error
//value as required. If PERM_ERROR is very small, the shaft will keep hunting left and right as the//analogRead() of the potentiometer pin keeps fluctuating slightly
#defineMAX_ANGLE180//we will allow our motor to turn by a maximum angle of 180 degrees
//**********************************
//Now we define a class that will control our DC motor....
//This motor must be running from a H bridge like L298 IC
//********************************************************************